Python Behave (BDD) Test Automation Framework for Web, API, & Browser Stack
Authors
Description
Python Behave Test Automation Framework is a behavior-driven development (BDD) framework designed for web, API, and browser stack testing. It leverages Python’s simplicity and readability along with the powerful Behave library to provide an efficient and structured approach to automated testing.
This framework aims to streamline the testing process by enabling testers and developers to write test scenarios in natural language using Gherkin syntax. It encourages collaboration between stakeholders by providing a common language for defining test cases and requirements, making it easier to understand and maintain test suites.
Features
- Behavior-Driven Development (BDD): Utilizes Gherkin syntax to define test scenarios in a human-readable format, fostering collaboration and communication between team members.
- Web Testing: Supports automated testing of web applications using Selenium WebDriver, allowing for UI testing across different browsers.
- API Testing: Provides capabilities for testing RESTful APIs using Python’s requests library, enabling validation of API endpoints and responses.
- Browser Stack Integration: Seamlessly integrates with BrowserStack for cross-browser and cross-platform testing, ensuring compatibility and consistency across various environments.
- Modular Structure: Organizes test code into reusable modules, making it easy to maintain and scale the test suite as the application evolves.
- Reporting and Logging: Generates detailed test reports in HTML format using Allure Framework, providing insights into test execution results, including logs, screenshots, and attachments.
- Configuration Management: Supports configuration management through JSON files, allowing for easy configuration of test data, environment settings, and browser capabilities.
- Continuous Integration (CI) Ready: Designed to be integrated with popular CI/CD tools like Jenkins, GitLab CI/CD, and GitHub Actions for automated test execution and reporting.
- Extensibility: Provides hooks and customizations for extending and enhancing test functionality, such as setting up test fixtures, teardown actions, and test data management.
- Scalability: Designed for scalability, allowing for the addition of new features, test cases, and integrations as the testing requirements grow.
Table of Contents
Pre-Requisite
- Python: 9.13
- Selenium: 4.7.2
- allure-behave: 2.10.0
- allure-python-commons: 2.10.0
- behave: 1.2.6
- webdriver-manager: 3.8.3
- allure-combine: latest
Technology_used_in_Framework
AUTOMATION:
Python
Selenium
Behave
webdriver-manager
allure-behave- ^2.10.0
allure-python-commons - ^2.10.0
allure-combine
FRAMEWORK DESIGN PATTERN :
Behavior Driven Development (BDD)
OS for Execution on Local:
OS for Execution on CI/CD:
Note:
Installation
To Clone this repository to a local directory
Commands to clone and run the test cases
The above command will clone this repository on your machine locally.
1)To run tests first need to install Selenium
2)Install Webdriver Manager
pip install webdriver-manager
3)Install Behave
4)Install Allure to generate the report
pip install allure-behave
pip install allure-python-commons
pip install allure-combine
Execution
5)To run all the feature file
behave Features -f allure_behave.formatter:AllureFormatter -o Report_Json
6)To run single feature file
behave Features/Bmi_Calculator.feature -f allure_behave.formatter:AllureFormatter -o Report_Json
7)To run test cases using Tag name from single feature file
behave Features/Bmi_Calculator.feature --tags=smoke -f allure_behave.formatter:AllureFormatter -o Report_Json
8)To run test cases using Tag name from all feature files
behave Features --tags=regression -f allure_behave.formatter:AllureFormatter -o Report_Json
Report_Generation
9)To generate HTML report folder from JSON report folder
allure generate Report_Json -o Report_Html --clean
10)To generate sharable HTML report
allure-combine Report_Html --dest Final_Report --auto-create-folders
Framework_Structure
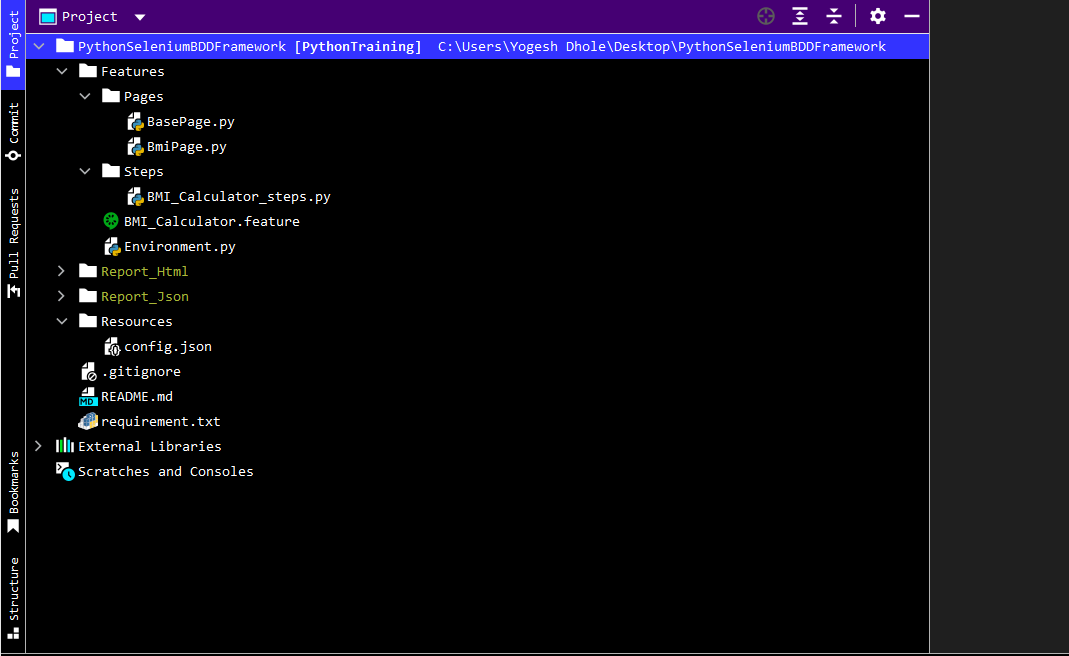
Features
- The feature file in the Python Behave Test Automation Framework follows the Gherkin syntax to define test scenarios in a human-readable format.
- It serves as a blueprint for writing test cases and requirements in a structured manner.
- Each feature file typically corresponds to a specific feature or functionality of the application under test.
Environment File (Hooks File)
- Environment.py:
- This file is used to initialize the browser with the help of before_scenario Hooks
- The file also launches the application url in before hooks for every test scenarios
- The file contains two execution mode i.e. local and using Browser Stack Cloud to execute the Test Cases
- The file has after_step hooks which captures the screenshots of every step mentioned in the feature File
- The after_scenario hook is used to close the page and the browser.
Pages
- Page files in the Python Behave Test Automation Framework represent the different pages or components of the application under test.
- Each page file corresponds to a specific page or component, making the test code modular and organized.
- These files typically contain page objects and methods that interact with the elements on the web pages.
- Features:*
- Modularization: Page files promote modularity by encapsulating page-specific functionality and interactions within separate modules, improving code readability and maintenance.
- Reusability: Page objects and methods defined in page files can be reused across multiple test scenarios, reducing duplication and promoting code reuse.
- Abstraction: Page files abstract the complexities of interacting with web elements, providing a clean and structured interface for test scripts to interact with the application’s UI.
Steps
- The step file contains the implementation of the test steps defined in the feature file.
- It uses Python code to automate the actions and validations specified in the Gherkin steps.
- Each step in the feature file maps to a corresponding step definition in the step file, where the actual testing logic is executed.
Resources
- The resources contain the config.json file which contains the key and value pairs of the most frequently used data in the framework. For example web url, LogIn credentials, Browser Stack credentials, etc.
- The resources folder include the driver files to run the test cases on particular browser.
Utils
- Utility files in the Python Behave Test Automation Framework contain helper functions, configurations, and reusable components that support the testing process.
- These files serve various purposes, such as data manipulation, logging, configuration management, and test setup.
- Features:
- Helper Functions: Utility files contain helper functions that perform common tasks such as data parsing, validation, and formatting, simplifying test script development.
- Configuration Management: Configuration files within utility files manage test data, environment variables, browser configurations, and other settings, providing flexibility and customization options.
- Logging and Reporting: Utility files include logging mechanisms for capturing test execution logs, errors, and debugging information. They also facilitate integration with reporting frameworks like Allure for generating comprehensive test reports.
- Reusable Components: Utility files encapsulate reusable components such as custom assertions, test fixtures, setup and teardown functions, and API request handlers, enhancing test script modularity and maintainability.
Resource
- The resources folder in the Python Behave Test Automation Framework contains essential resources used during test execution, such as test data, configuration files, JSON files, and other assets required for testing.
- Features:
- Test Data Management: The resources folder stores test data files in various formats (e.g., JSON, CSV, Excel) for parameterizing test cases and conducting data-driven testing.
- Configuration Files: Configuration files within the resources folder manage environment-specific configurations, including URLs, credentials, timeouts, and other settings needed for test execution.
- Assets and Attachments: The resources folder may include assets such as images, documents, and attachments used in test scenarios or included in test reports for comprehensive documentation and analysis.
Generated_Reports
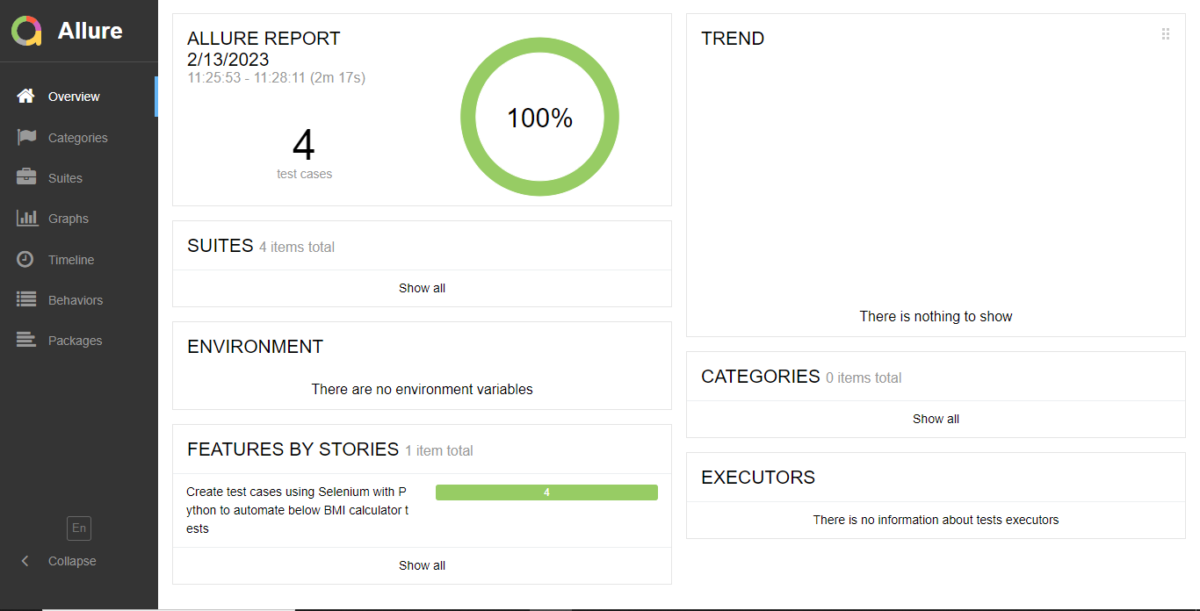
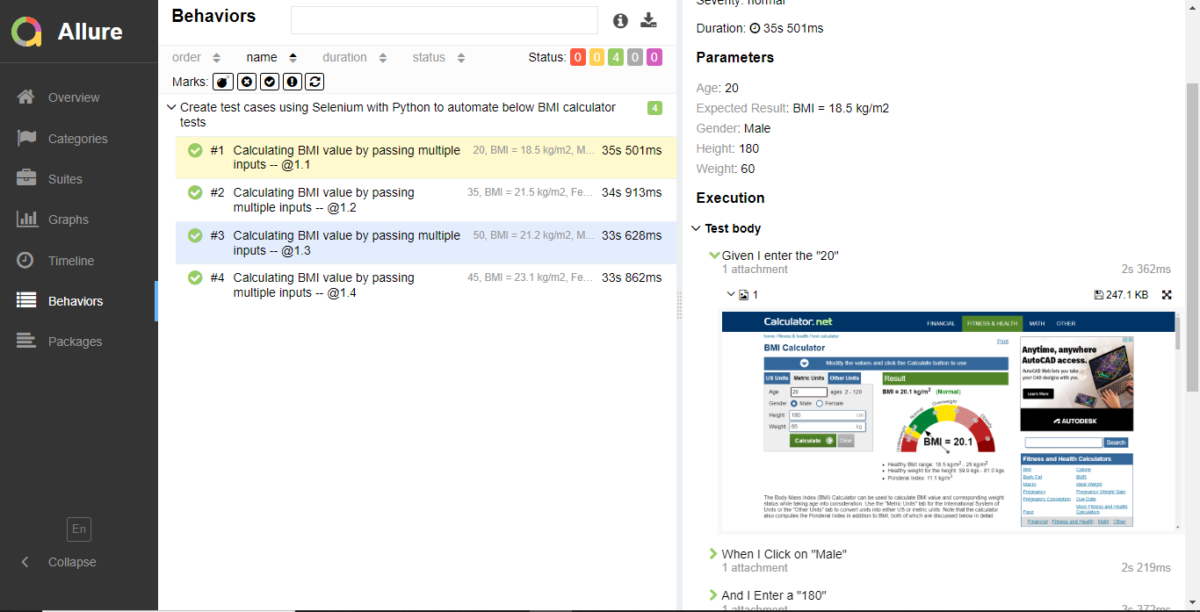